Lua (/ ˈ l uː ə / LOO-ə; from Portuguese: lua meaning moon) is a lightweight, high-level, multi-paradigm programming language designed primarily for embedded use in applications. Lua is cross-platform, since the interpreter of compiled bytecode is written in ANSI C, and Lua has a relatively simple C API to embed it into applications.
No CS experience required
CS First empowers every teacher to teach computer science with free tools and resources.
Fun hands-on learning
Students learn through video tutorials and block-based coding in Scratch.
Always free
CS First is totally free — any number of students, all materials, as many lessons as you want.
A curriculum for every classroom
Students learn through video-based lessons, with different themes like sports, art, and game design.
Manage class progress with a dashboard
Community highlights
See what teachers and students are doing with CS First
A middle school student from California learns coding using the 'Create your own Google Logo' lesson
'If it wasn't for CS First, our small, rural school wouldn't be leading the way in introducing students to Computer Science in Nebraska'
Kyleigh Lewis
Middle/High School Teacher
An 'eggcelent' Scratch Project created from the 'An Unusual Discovery' lesson
Sign in to manage student progress with your dashboard, save your students' work, and schedule your first class.
This is Part 2 of my Integrating Lua into C++ Tutorial series.
For this part of the tutorial series, we will be discussing on how to interact with classes and objects, both in Lua and C++.
Using a C++ class in Lua
First off, let’s bind a simple C++ class so that we can use the class in our lua code. You can name your class anything, but I’m going to use something simple, ‘CppObject’.
Create a class with the following interface and implementation:
CppObject.hpp
This is a simple c++ class with a function that prints out “Test1Function1 Called!” when called.
This code helps to bind the C++ object into a Lua object with the alias ‘CppObject.
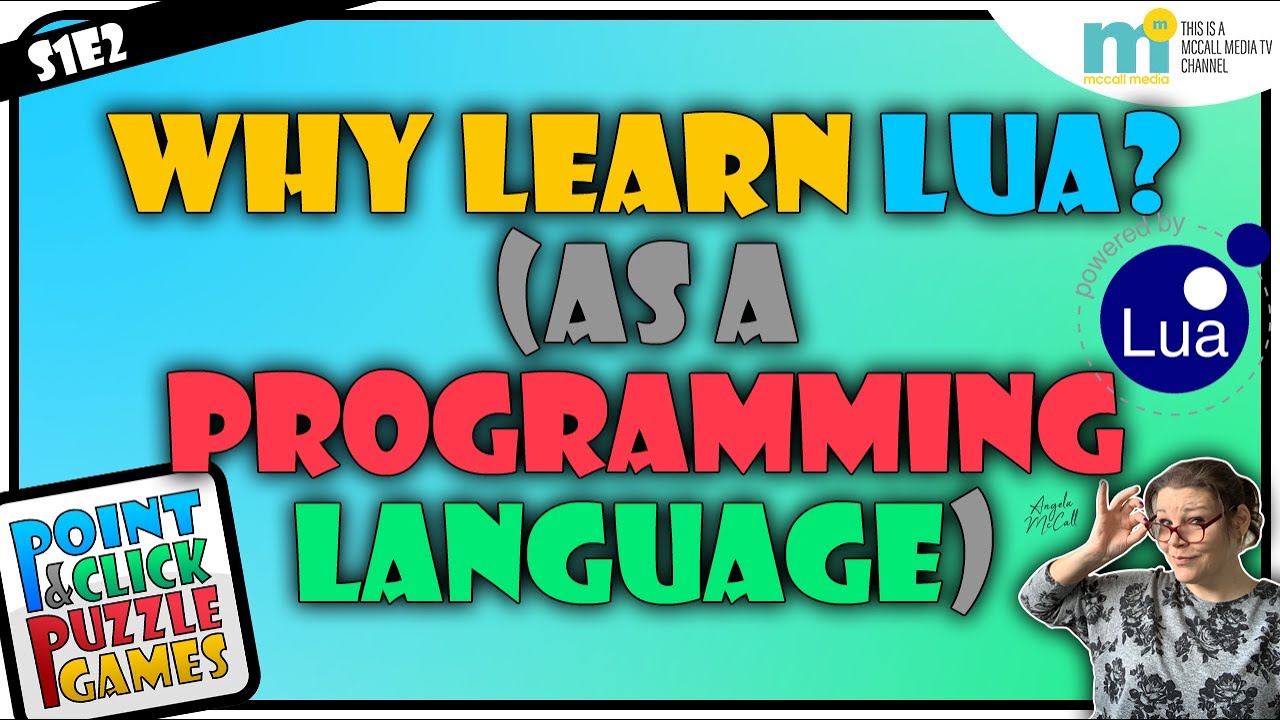
Lets dissect the code line by line.
state.new_usertype<CppObject>('CppObject', 'TestFunction1', &CppObject::TestFunction1);
This line defines the new Lua Usertype and also defines the member function ‘TestFunction1’ to specify that the class contains this function.
state.do_string('cppObj = CppObject:new() cppObj:TestFunction1()');
In this line, we create a new object ‘cppObj’ of type ‘CppObject’ and invoked it’s member function.
If all goes well, you should printed:
TestFunction1 Called!
Congratulations! You now know how to play around with C++ Objects in your lua code. If you are really interested in learning more about this, The tutorials over in the Sol2 github page really covers this extensively.
Using a Lua class in Lua
Before we go over using a Lua class in C++, let’s first do it in Lua.
Learn Lua Or C++ First
The Lua language does not have any implementation of classes but they do have something called metatable that allows you to do really cool stuffs. One of them is to implement a class system. For this, we will be using a library called middleclass. Middleclass is an OOP library that I really like to use. It’s very lightweight, and easy to use.
I will only be covering the basics, so head over to their github page for more info.
For starters, download the middleclass file and then add it into your scripts folder. I’ll show you how to set it up later.
For now, go to your scripts folder and create a new lua file with the following code:
TestClass.lua
The code above defines the class that we will be using, ‘TestClass’.
The initialize function will be called whenever a TestClass object is created. You can say that it’s the constructor of the class.
state['package']['path'] = (package_path + ';scripts/middleclass.lua').c_str();
Here, I initialized the middleclass library by adding it into the luastate”s package path.
state.do_file('scripts/TestClass.lua');
I then ran the ‘TestClass.lua’ file that defines my TestClass Lua class.
state.do_string('testObj = TestClass:new() testObj.TestFunctionCall()');
I then created a TestClass object and called it’s member function ‘TestFunctionCall’
If everything went well, you should be printing this on the console:
TestClass Created!
TestClass TestFunction Called!
This is just a basic functionality for using MiddleClass together with Sol2. I hope you find it useful.
Using a Lua class in C++
Now we get to the more interesting part of the tutorial, using a lua object in c++. I find that the best way for this is to create a wrapper class.
You actually do not need to create a wrapper class like ScriptObject as you can just save it into a sol::table but I would recommend making one to make your life easier.
ScriptObject.hpp
Whew this is rather long. This class omits out quite a few functions for it to be usable, but it contains the bare-bones of what we need for the tutorial. I wanted to keep it simple. Don’t worry though, I’ll share the full class implementation later.
This is the non-default constructor of the ScriptObject.
This function creates a Lua object of type ‘luaClassName’ and gives them a unique name for use in the luaState. The name is then saved in m_scriptVarName.
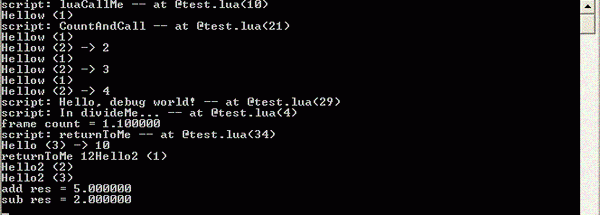
m_luaObjectData = (*m_pLuaState)[m_scriptVarName];
If you remember the previous tutorial, then this should be rather familiar. Here, we save the lua object into m_luaObjectData for easy access.
m_pLuaState->script(luaScript, [&isValidCreation](lua_State* state, sol::protected_function_result res) { isValidCreation = false; return res; });
The most complex part about this function would most probably be in this line. I created this lambda function to suppress an exception being thrown if the lua code called is not valid. In this case, it would usually be called if the Lua-class of the object you are trying to create is not valid.
If an exception is thrown, we will set isValidCreation to false, and then stop with the creation of the object.
If m_initialized boolean is set to true at the end of the function, it would mean that your object is successfully created.
This helper function helps us call the lua object’s member functions.
In the destructor, we set the object to nil and then let the garbage collection do all the work.
Alright, so we have already defined our ScriptObject wrapper class. So now let’s try using it.
So here we create our ScriptObject object and then call the “TestFunctionCall” function. The behaviour is totally the same as the previous example.
If everything went well, same as before, you should be printing this on the console:
TestClass Created!
TestClass TestFunction Called!
Summary
This part of the tutorial is where you start having the basic knowledge on OOP on Lua, as well as handling classes in both Lua and C++ codes.
Codes
Learn Lua Or C First Bank
CppObject.hpp
ScriptObject.hpp
ScriptObject.cpp
Main.cpp
Previous (Part 1b: The Basics) | Next(Part 2.5: Adding Templates to ScriptObject Class)