- Roblox Lua C++ Source Code
- Roblox Lua Source
- Roblox Lua Course Free
- Roblox Lua Course
- Roblox Lua Course Codakid
In this short tutorial I'll show how to run Lua programs from C and C++ and howto expose functions to them. It's easy!
Update: The code in this post has been updated for Lua 5.2.4. I haven'tchecked if the Lua 5.3 C API is backwards-compatible with 5.2. All the codehere is available on GitHub.
The first program will just create a Lua state object and exit. It will be ahybrid between C and C++. Since the two languages must include different files,we need to discern between them by checking for the existence of the__cplusplus
macro.
Roblox is a global platform that brings people together through play. Roblox is ushering in the next generation of entertainment. Imagine, create, and play together with millions of people across an infinite variety of immersive, user-generated 3D worlds. Closed Source Modules (Private Modules) are just what they say they are. Open Source is where your source code is clearly visible and can be copied. Closed Source is where its just closed off, and no one can obtain your source code. This was a feature Roblox supported, up until February 2019. Obfuscation isn’t really closed source.
Notice that I'm being explicit about which version of Lua I'm using in thecode. If you trust that the Lua developers care about compatibility, you canjust #include <lua.hpp>
and so on directly.
The purpose of the program is just to make sure that we can compile, link andrun it without errors.
You need to let the compiler know where it can find the include files and theLua shared library. The include files are usually located in/usr/local/include
and the library files in /usr/local/lib
. Search yoursystem directories if needed. To compile the above program, pass thedirectories with -I
and -L
, respectively.
You may swap out g++
with llvm-g++
, or just c++
, depending on yourcompiler. If you're using a C compiler, use gcc
or llvm-gcc
— butremember to rename the file to first.c
.
Now try to run the program to make sure it doesn't segfault:
This one worked just fine.
Executing Lua programs from a host
The next step is to execute Lua programs from your C or C++ code. We'll createthe Lua state object as above, load a file from disk and execute it.
Put this into runlua.cpp
or runlua.c
:
You can reuse the compilation arguments from above:
or
Running Lua programs
Let's test this with some Lua programs. The first one prints the Lua versionand exits.
You may want to double-check that it works by running lua hello.lua
. It maynot be important for this trivial program, but can become important when youtry more advanced ones.
Now try it with runlua
:
Roblox Lua C++ Source Code
You can even run bytecode-compiled programs:
Roblox Lua Source
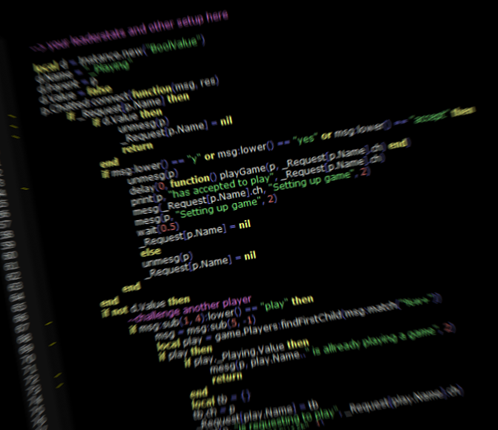
Roblox Lua Course Free
We should also check that the error handling works. Put some garbage in a filecalled error.lua
, for example
Running it produces
Calling C functions from Lua
It gets very interesting when Lua programs call back to your C or C++functions. We'll create a function called howdy
that prints its inputarguments and returns the integer 123.
To be on the safe side, we'll declare C linkage for the function in the C++version of the program. This has to do with name mangling,but in this case, it really doesn't matter: Lua just receives a pointer to afunction, and that's that. But if you start using dynamic loading of sharedlibraries through dlopen
and dlsym
, this will be an issue. So let's do itcorrect from the start.
Roblox Lua Course
Copy the above program into a file called callback.cpp
and add the howdy
function.
We have to pass the address of this function to Lua along with a name. Put thefollowing line somewhere between the call to lua_newstate
andluaL_loadfile
:
Create a test program called callback.lua
Compile and test it
I told you it was easy!
What next?
Read the Lua C APIReference. You've learned enough now to get going with it. Did you see mynote about clearing the stack in howdy
? You may want to investigate that.
Find out how to integrate Lua closures with your C functions.
If you want to hide or catch console output from Lua, you need to figure thatout as well. I once did it by trapping io.write()
; I copied its code fromlualib.c
and changed io_write
to point to my own function. There isprobably a better way to do it, though. Doing so is useful for things like gameprogramming.
Use RAIIor smart pointers to manage resources like lua_State
.
Roblox Lua Course Codakid
I also strongly recommend to try out LuaJIT.Calling into your functions there is even easier, using LuaJIT's foreignfunction library. I'll write a blog post on how todo that as well. In short, just create ordinary C functions, compile as ashared library, copy their signatures into pure Lua source code and hook themup with LuaJIT's FFIlibrary.
LuaJIT runs between 10-20 and up to 135 times faster than interpreted Lua, soit's definitely worth it.